Note! The following instruction is dedicated for the second generation HTTP Gate module!
The following instruction for integration with Tedee lock is based on the information provided on:
https://tedee-tedee-api-doc.readthedocs-hosted.com/en/latest/index.html.
To integrate Grenton system with Tedee lock, please follow the steps described below:
1. Create following User features on Gate_HTTP.
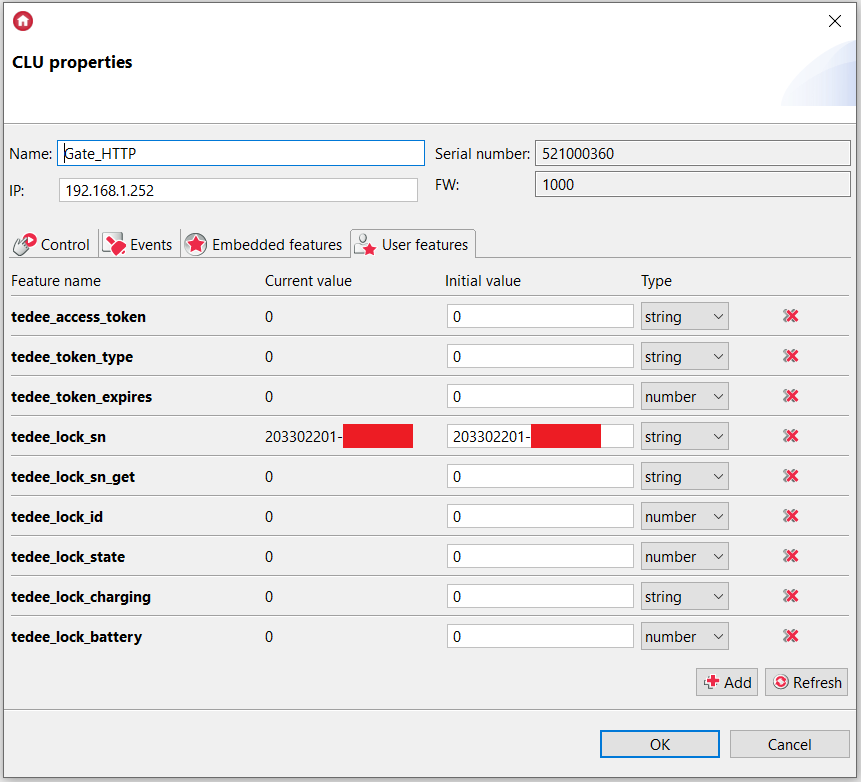
Note! The value of the tedee_lock_sn feature is the serial number of your lock. This information is available in the dedicated application: My devices-> Door Lock -> Lock -> Settings -> Information.
2. Create a HttpRequest virtual object.
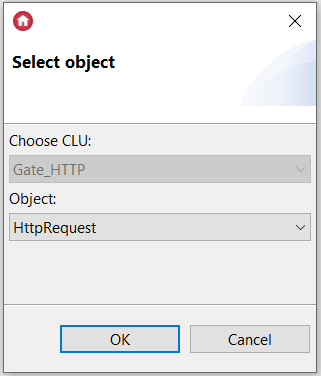
3. Set the TedeeLock_AuthorizationKey virtual object’s properties as follows. This configuration allows retrieving basic information about the lock (i.a. access key).
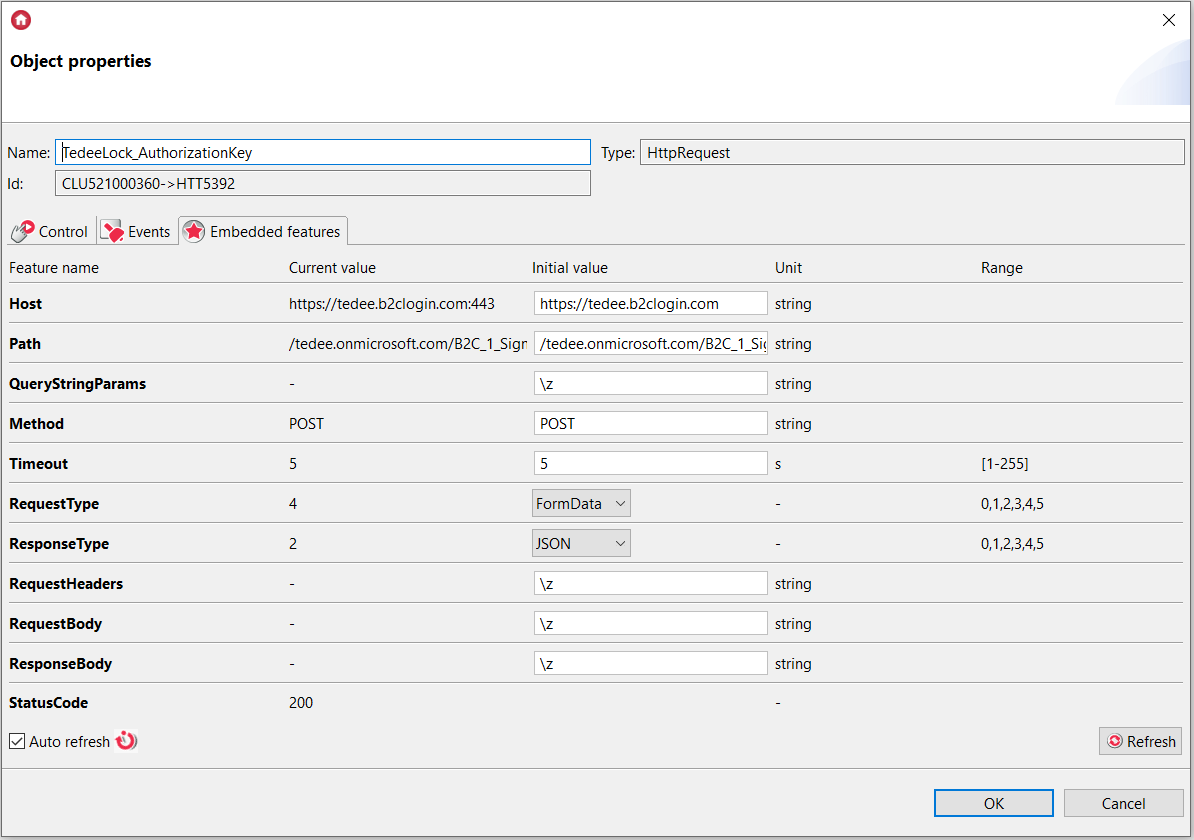
Where:
Host: https://tedee.b2clogin.com
Path: /tedee.onmicrosoft.com/B2C_1_SignIn_Ropc/oauth2/v2.0/token
QueryStringParams: \z
4. Create a Tedee_AuthorizationKey_req script.

Note! The username (MAIL) and password (PASSWORD) must be replaced with the data used to log in to the dedicated application (according to URL encoding).
Note! The @ sign in the email address should be replaced by %40.
local get_key = {"&grant_type=password&client_id=02106b82-0524-4fd3-ac57-af774f340979&scope=openid+02106b82-0524-4fd3-ac57-af774f340979&response_type=token&username=MAIL&password=PASSWORD"}
Gate_HTTP->TedeeLock_AuthorizationKey->SetRequestBody(get_key)
Gate_HTTP->TedeeLock_AuthorizationKey->SendRequest()
5. Create a Tedee_AuthorizationKey_resp script.

if(Gate_HTTP->TedeeLock_AuthorizationKey->StatusCode==200) then
local resp = Gate_HTTP->TedeeLock_AuthorizationKey->ResponseBody
Gate_HTTP->tedee_access_token = resp.access_token
Gate_HTTP->tedee_token_type = resp.token_type
Gate_HTTP->tedee_token_expires = resp.expires_in
end
6. Assign this script to OnResponse event of the TedeeLock_AuthorizationKey virtual object.
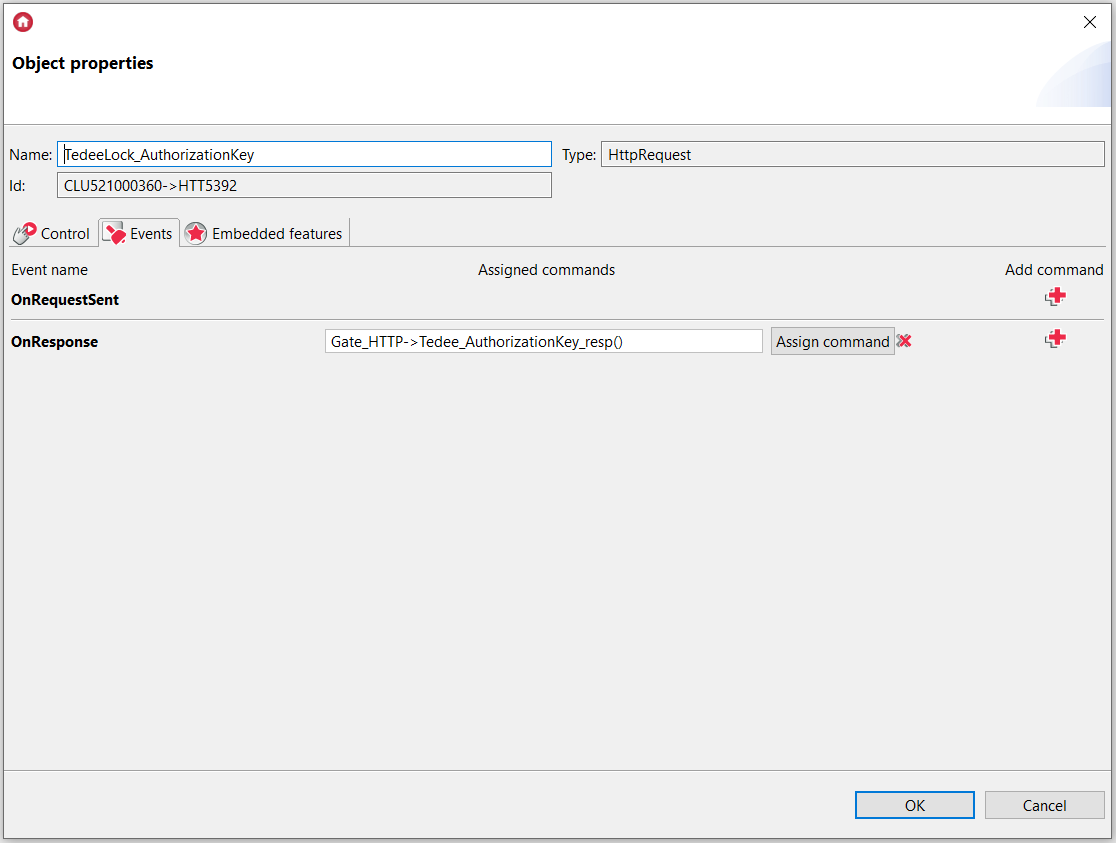
7. Send the configuration to CLU and run the Tedee_AuthorizationKey_req script. User features will take appropriate values.
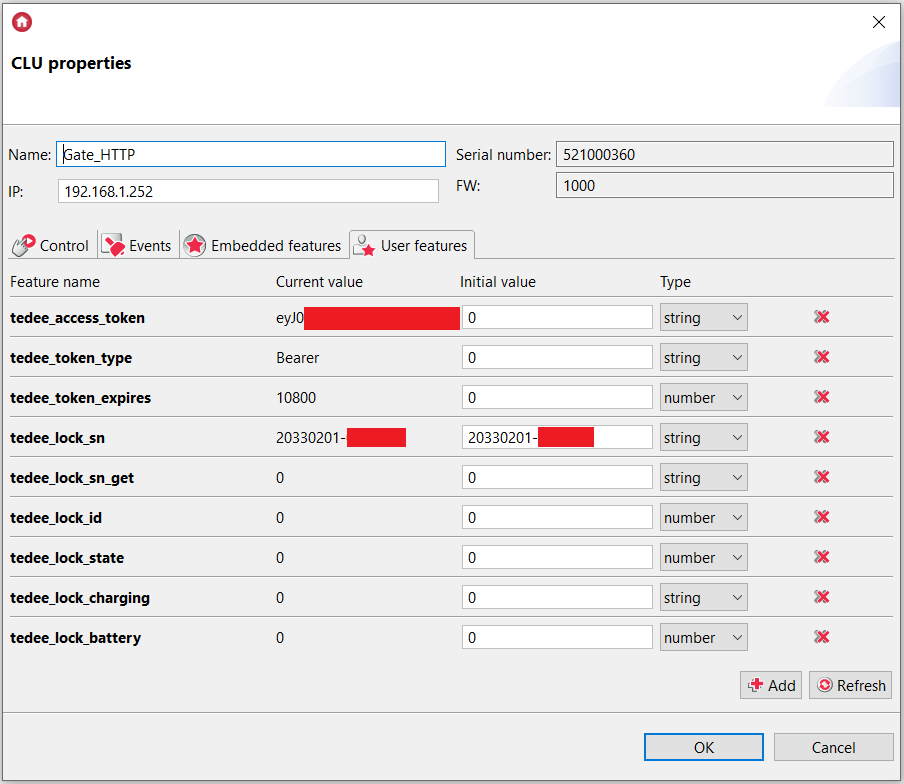
8. Create a HttpRequest virtual object - TedeeLock_DeviceID – which allows retrieving the lock’s ID.
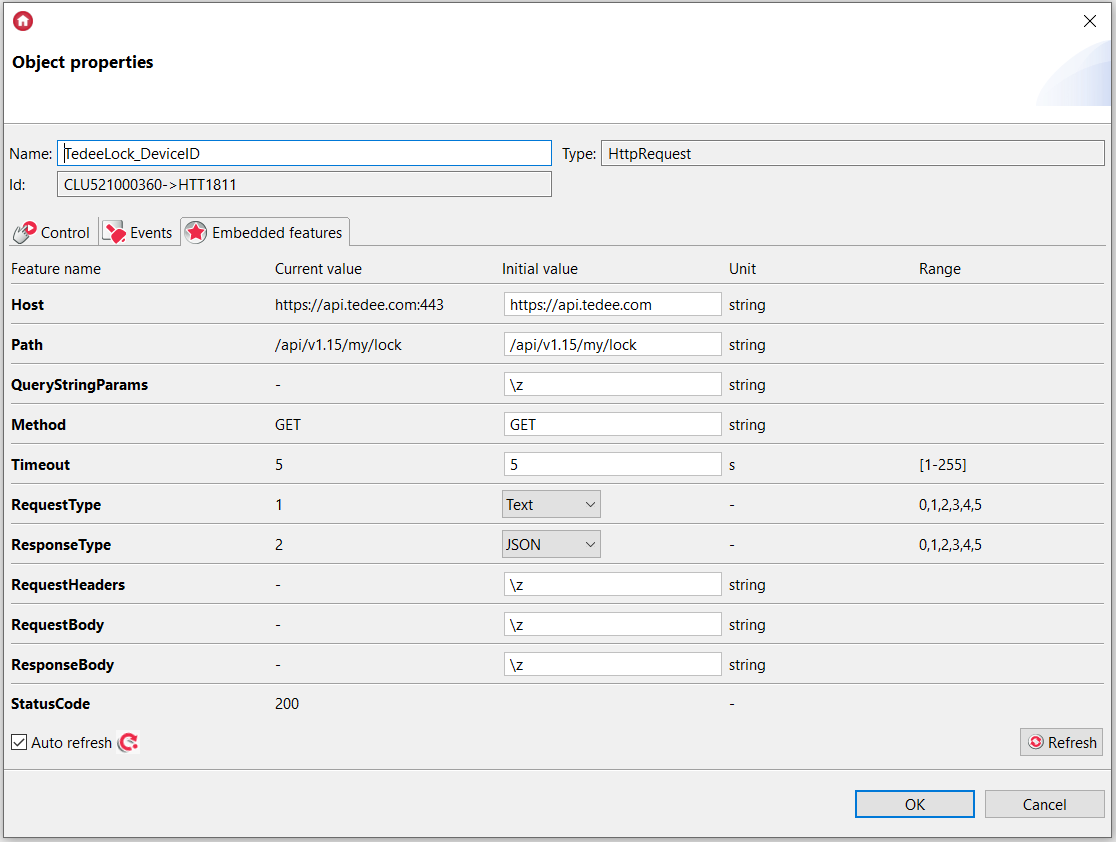
Where:
Host: https://api.tedee.com
Path: /api/v1.15/my/lock
QueryStringParams: \z
9. Create a Tedee_DeviceID_req script.

local key = "Authorization: Bearer " .. Gate_HTTP->tedee_access_token .. "\r\n"
Gate_HTTP->TedeeLock_DeviceID->SetRequestHeaders(key)
Gate_HTTP->TedeeLock_DeviceID->SendRequest()
10. Create a Tedee_DeviceID_resp script.
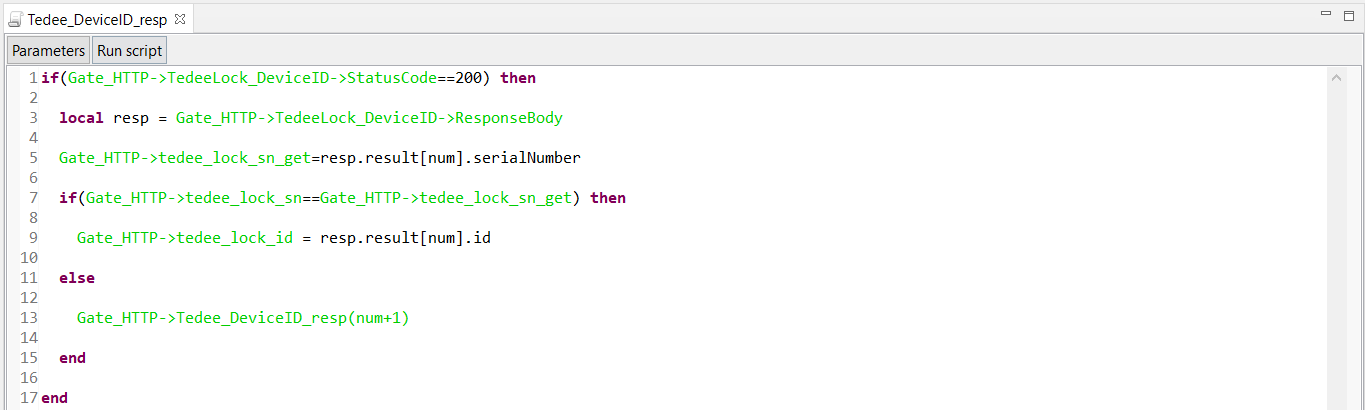
if(Gate_HTTP->TedeeLock_DeviceID->StatusCode==200) then
local resp = Gate_HTTP->TedeeLock_DeviceID->ResponseBody
Gate_HTTP->tedee_lock_sn_get=resp.result[num].serialNumber
if(Gate_HTTP->tedee_lock_sn==Gate_HTTP->tedee_lock_sn_get) then
Gate_HTTP->tedee_lock_id = resp.result[num].id
else
Gate_HTTP->Tedee_DeviceID_resp(num+1)
end
end
Where num feature is a parameter of this script.
Note! One Bridge and one Tedee lock were used in the example shown.
11. Assign Tedee_DeviceID_resp script with num parameter equal 1 to OnResponse event of the TedeeLock_DeviceID virtual object.
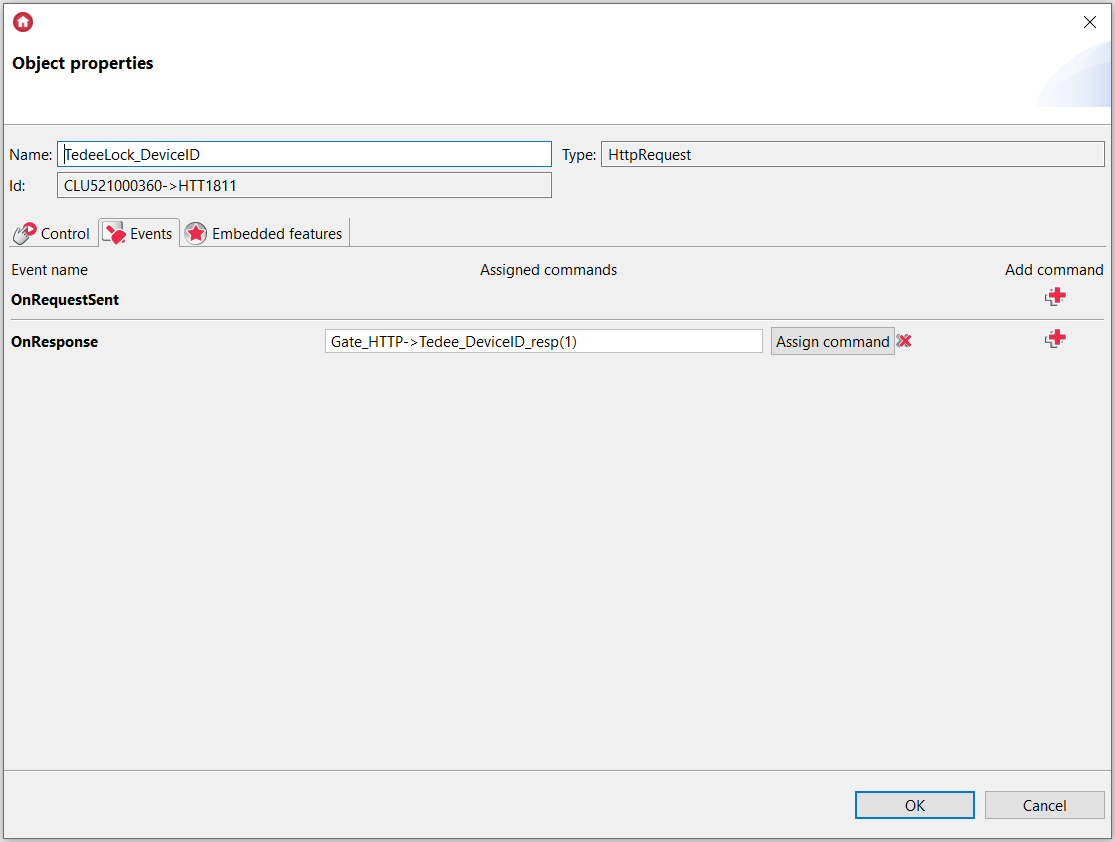
12. Send the configuration to CLU and run the Tedee_AuthorizationKey_req and Tedee_DeviceID_req scripts. User features will take appropriate values.
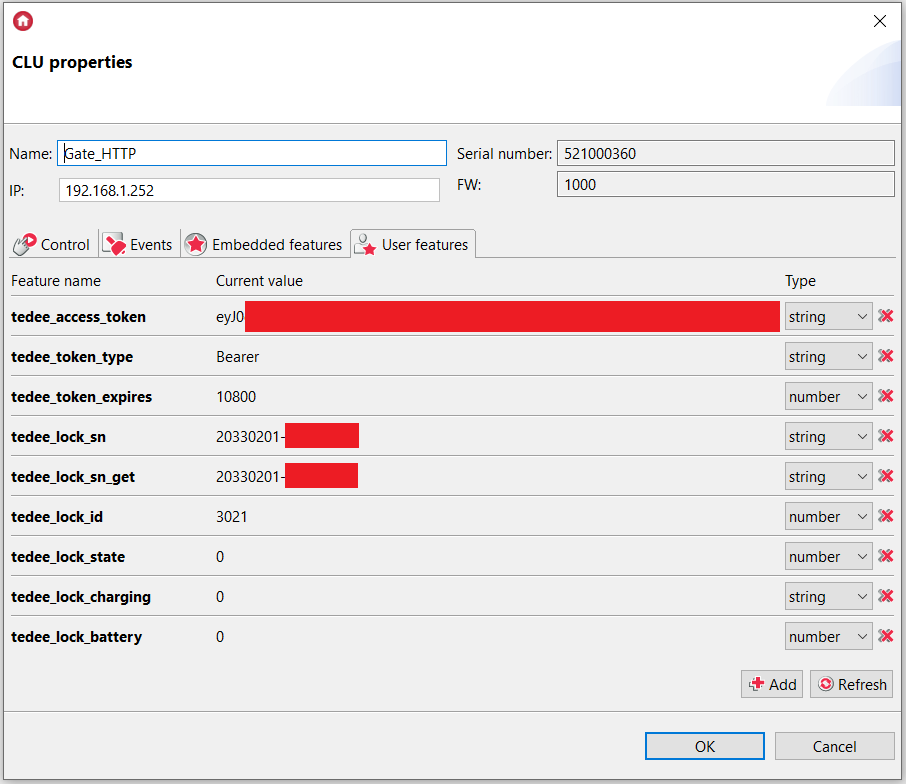
13. Create a HttpRequest virtual object - TedeeLock_LockID – which allows retrieving information about the lock (i.a. lock battery level).
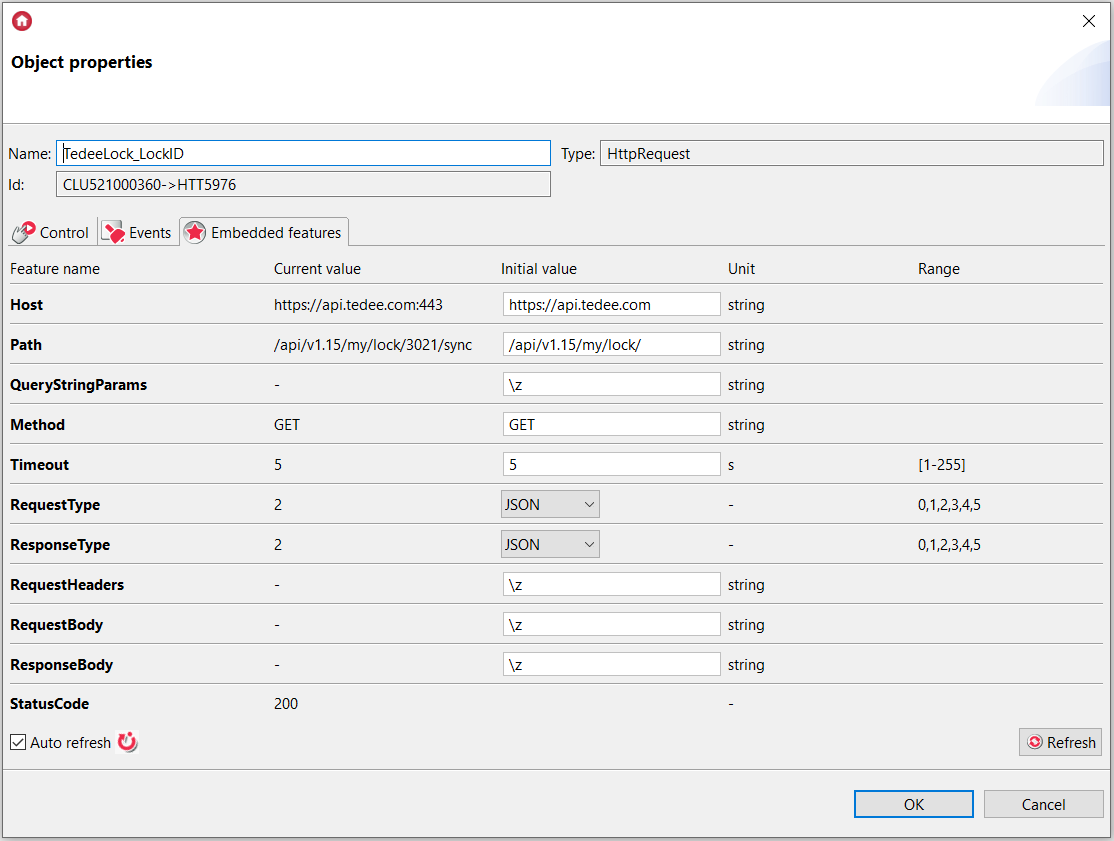
Where:
Host: https://api.tedee.com
Path: /api/v1.15/my/lock/
QueryStringParams: \z
14. Create a Tedee_LockID_req script.

local path_1 = "/api/v1.15/my/lock/" .. Gate_HTTP->tedee_lock_id .. "/sync"
Gate_HTTP->TedeeLock_LockID->SetPath(path_1)
local key = "Authorization: Bearer " .. Gate_HTTP->tedee_access_token .. "\r\n"
Gate_HTTP->TedeeLock_LockID->SetRequestHeaders(key)
Gate_HTTP->TedeeLock_LockID->SendRequest()
15. Create a Tedee_LockID_resp script.

if(Gate_HTTP->TedeeLock_LockID->StatusCode==200) then
local resp = Gate_HTTP->TedeeLock_LockID->ResponseBody
Gate_HTTP->tedee_lock_state = resp.result.lockProperties.state
Gate_HTTP->tedee_lock_charging = resp.result.lockProperties.isCharging
Gate_HTTP->tedee_lock_battery = resp.result.lockProperties.batteryLevel
end
16. Assign this script to OnResponse event of the TedeeLock_ LockID virtual object.
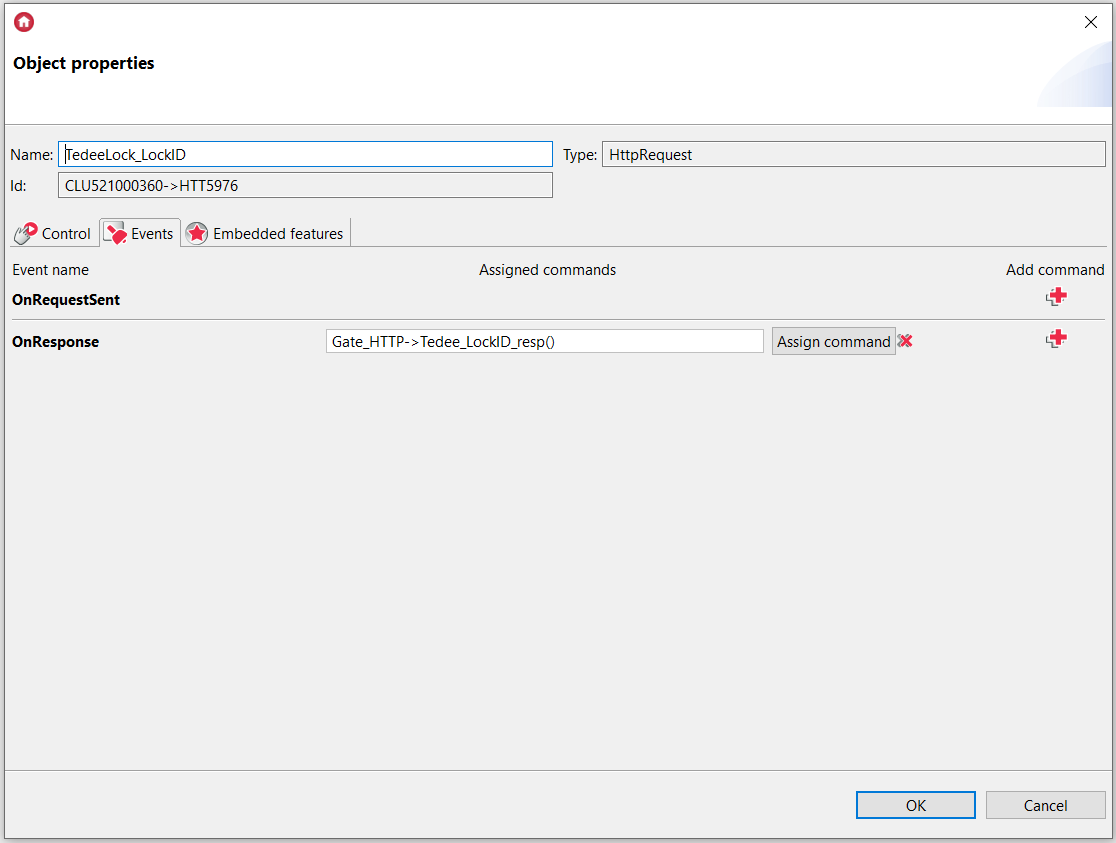
17. Send the configuration to CLU and run the Tedee_AuthorizationKey_req and Tedee_DeviceID_req and Tedee_LockID_req scripts. User features will take appropriate values.
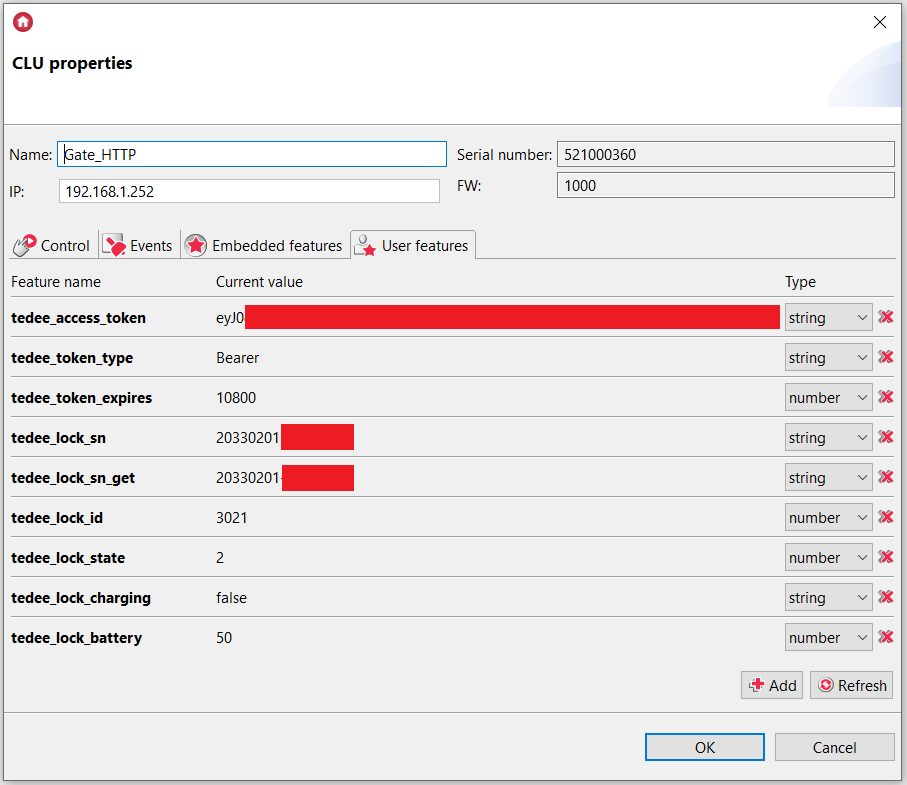
18. Create a HttpRequest virtual object - TedeeLock_Open – which allows to open the lock.
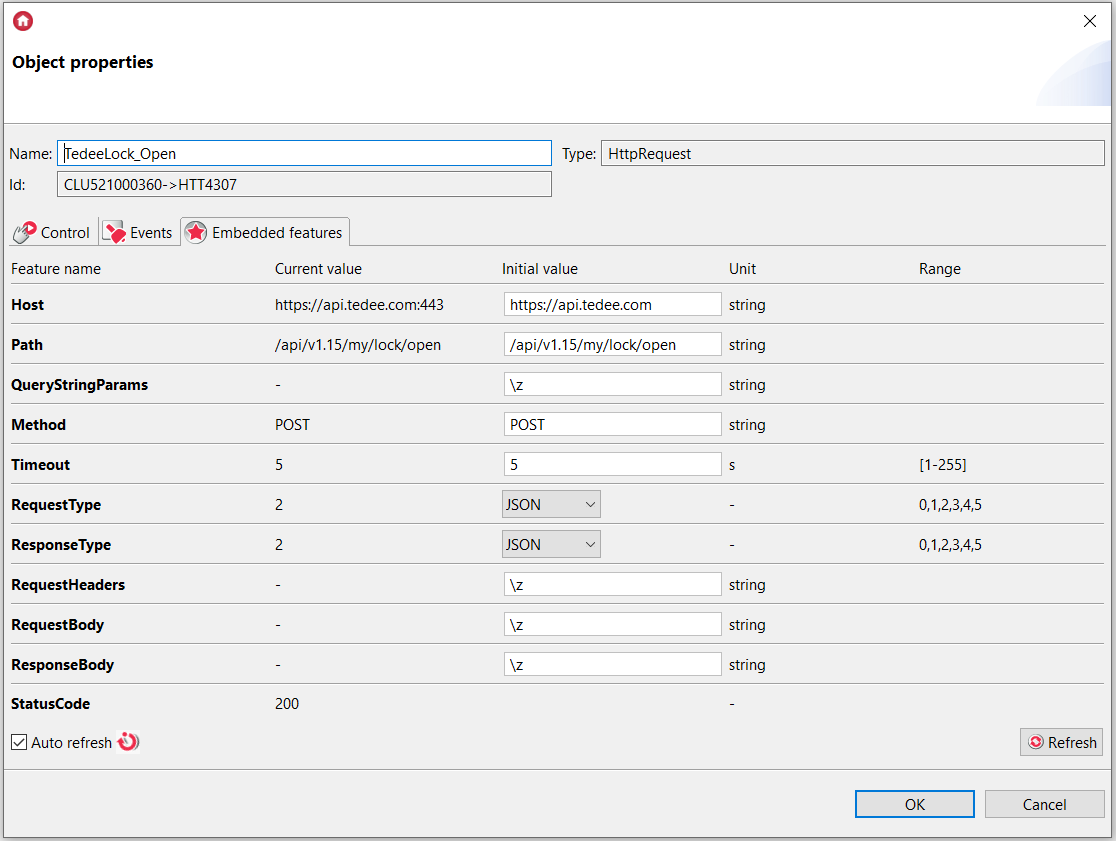
Where:
Host: https://api.tedee.com
Path: /api/v1.15/my/lock/open
QueryStringParams: \z
19. Create a Tedee_ Open_req script. After sending configuration to CLU, this script will open the lock.

local body_1 = {deviceId = Gate_HTTP->tedee_lock_id}
Gate_HTTP->TedeeLock_Open->SetRequestBody(body_1)
local key = "Authorization: Bearer " .. Gate_HTTP->tedee_access_token .. "\r\n"
Gate_HTTP->TedeeLock_Open->SetRequestHeaders(key)
Gate_HTTP->TedeeLock_Open->SendRequest()
20. Create a HttpRequest virtual object - TedeeLock_Close – which allows to close the lock.
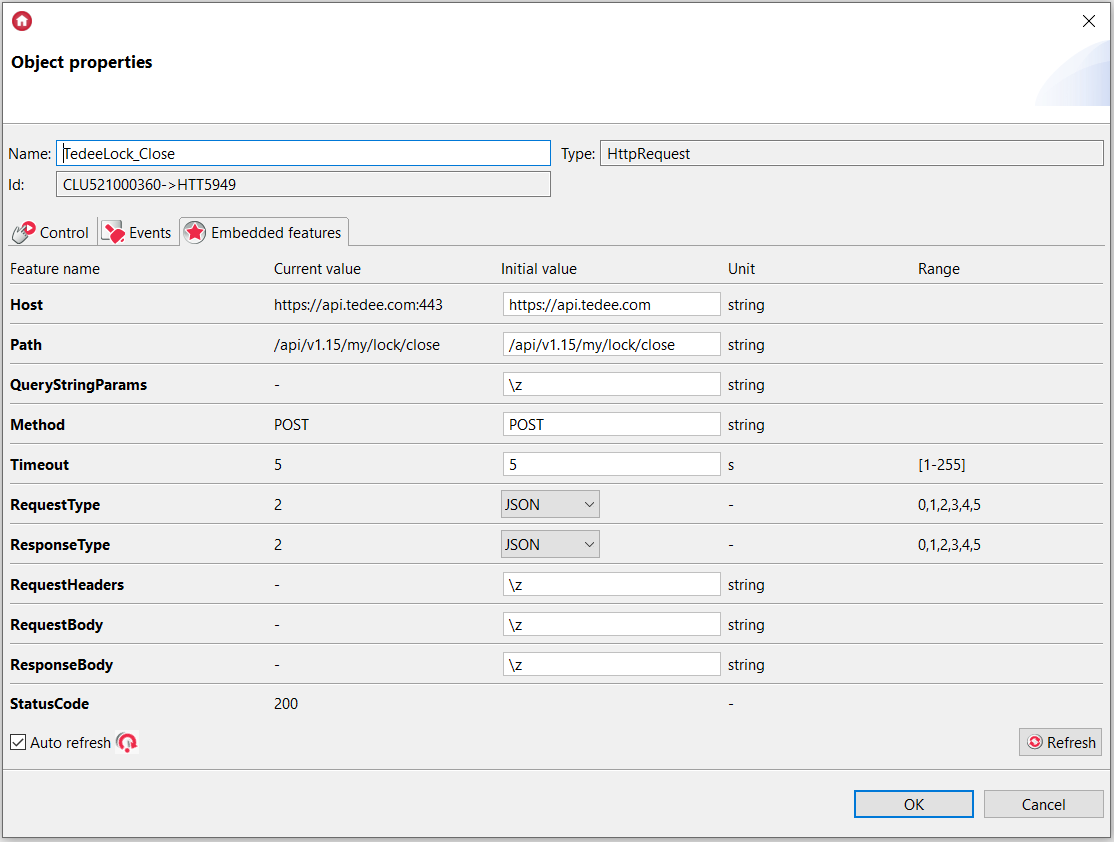
Where:
Host: https://api.tedee.com
Path: /api/v1.15/my/lock/close
QueryStringParams: \z
21. Create a Tedee_Close_req script. After sending configuration to CLU, this script will lock the lock.

local body_1 = {deviceId = Gate_HTTP->tedee_lock_id}
Gate_HTTP->TedeeLock_Close->SetRequestBody(body_1)
local key = "Authorization: Bearer " .. Gate_HTTP->tedee_access_token .. "\r\n"
Gate_HTTP->TedeeLock_Close->SetRequestHeaders(key)
Gate_HTTP->TedeeLock_Close->SendRequest()
22. Create a HttpRequest virtual object - TedeeLock_ PullSpring – which allows to pull the spring.
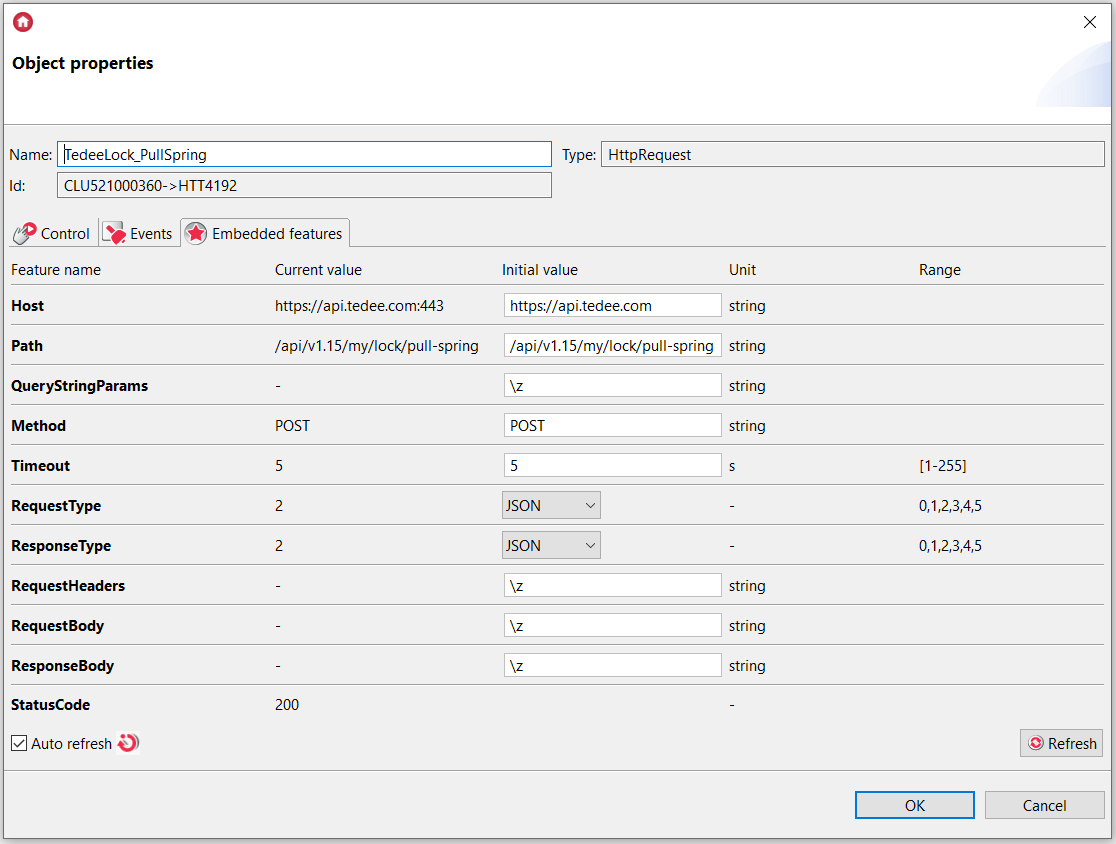
Where:
Host: https://api.tedee.com
Path: /api/v1.15/my/lock/pull-spring
QueryStringParams: \z
23. Create a Tedee_ PullSpring_req script. After sending configuration to CLU, this script will pull the spring.

local body_1 = {deviceId = Gate_HTTP->tedee_lock_id}
Gate_HTTP->TedeeLock_PullSpring->SetRequestBody(body_1)
local key = "Authorization: Bearer " .. Gate_HTTP->tedee_access_token .. "\r\n"
Gate_HTTP->TedeeLock_PullSpring->SetRequestHeaders(key)
Gate_HTTP->TedeeLock_PullSpring->SendRequest()
24. At the end, the call of Tedee_AuthorizationKey_req script assign to OnInit event on Gate_HTTP.
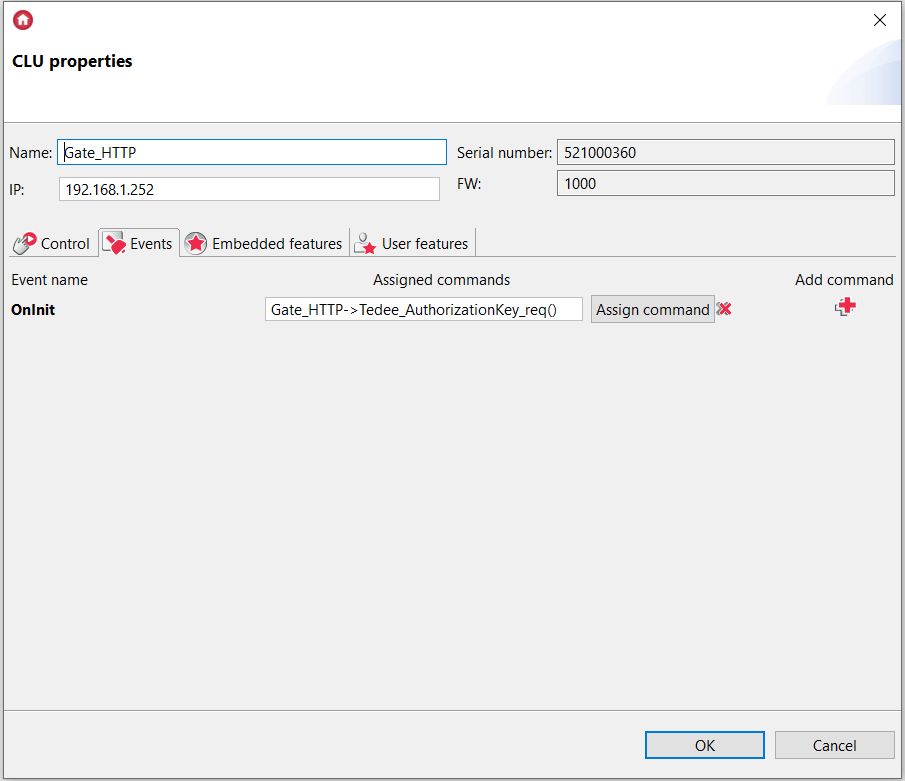
Note! The token expires after defined time (tedee_token_expires user feature), thus the Tedee_AuthorizationKey_req script requires to be called cyclically, for example by using a Calendar virtual object.
25. The call of Tedee_DeviceID_req script add in Tedee_AuthorizationKey_resp script.
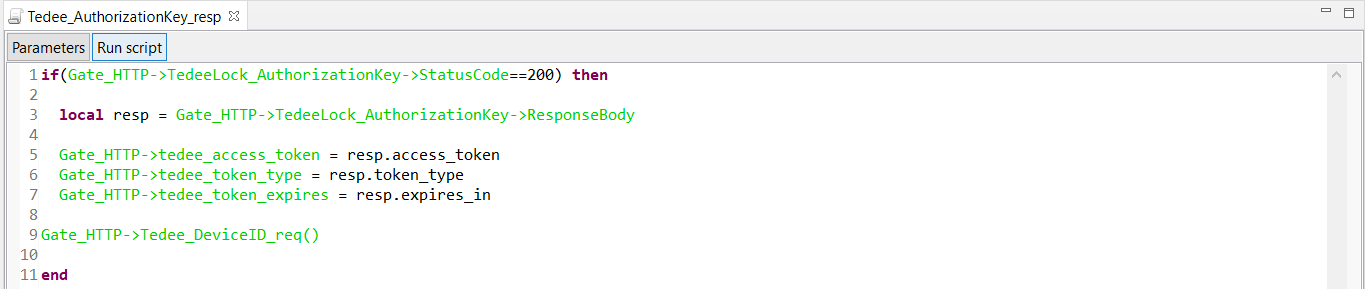
26. The call of Tedee_LockID_req script add in Tedee_DeviceID_resp script.
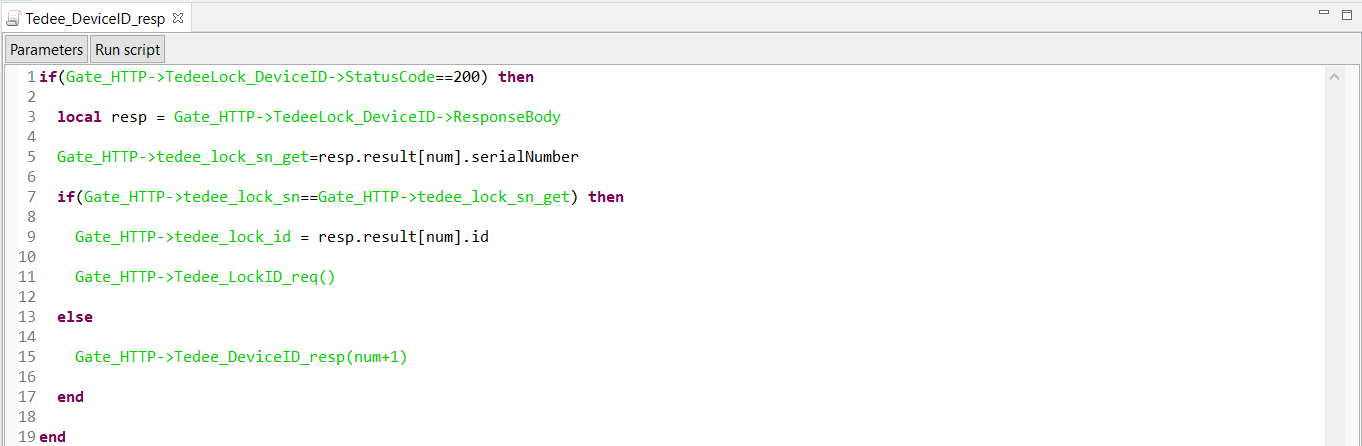
27. The configuration prepared in that way, send to Gate_HTTP module.
The way to control a Tedee lock using the myGrenton app is presented in the following article: Control with Tedee Lock.
